How to export CSV using csv module in NodeJS
01 Aug 2024 - 3 min read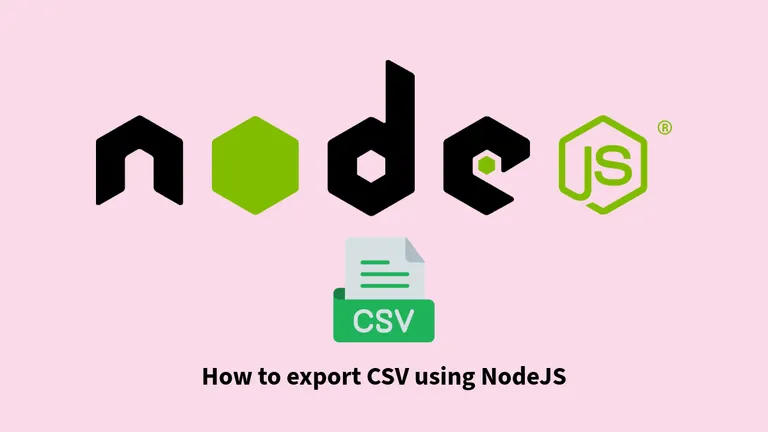
The csv module in Node.js is a versatile library that provides comprehensive functionality for parsing and writing CSV files. It offers features like support for various delimiters, encoding, and streaming capabilities, making it suitable for handling both small and large datasets efficiently.
Here’s a detailed guide on how to use the csv module to write CSV files in Node.js.
Table of Contents
- Step 1: Install the
csv
Module - Step 2: Write CSV Files Using
csv-stringify
- Advance: Using
csv
Module for Streaming Data - Conclusion
Step 1: Install the csv
Module
First, you need to install the csv
module using npm. This package includes multiple utilities, including csv-stringify
for writing CSV data.
npm install csv
yarn add csv
Step 2: Write CSV Files Using csv-stringify
The csv-stringify
utility from the csv
module is specifically designed for converting data into CSV format. It allows you to write data to a CSV file easily.
Here’s a simple example to demonstrate writing a CSV file using the csv module:
import { stringify } from "csv"import fs from "fs"import util from "util"
const main = async () => { // Sample data to be written to CSV const records = [ ]
// Create a writable stream for the CSV file const writableStream = fs.createWriteStream("./output.csv")
// Use the stringify function from csv module const output = await util.promisify(stringify)(records, { header: true })
// Write to the file writableStream.write(output, "utf8", () => { console.log("CSV file was written successfully") })}
main()
Advance: Using csv
Module for Streaming Data
When dealing with large datasets, streaming is a more efficient way to write CSV files. The csv
module supports streaming through the Stringifier
class, allowing you to write data in chunks.
Here’s how you can write CSV data using streams:
import { stringify } from "csv"import fs from "fs"
// Sample data to be written to CSVconst data = []
// Define the columns for the CSVconst columns = ["name", "age", "email"]
// Create a write stream for the output fileconst writeStream = fs.createWriteStream("./output.csv")
// Create a CSV stringifier instanceconst stringifier = stringify({ header: true, columns: columns })
// Pipe the stringifier output to the write streamstringifier.pipe(writeStream)
// Write each record to the stringifierdata.forEach((record) => stringifier.write(record))
// End the stringifier to finalize the filestringifier.end(() => { console.log("CSV file was written successfully with streaming")})
Conclusion
The csv
module in Node.js offers a flexible and efficient way to write CSV files, whether you are handling small datasets or large streaming data. By utilizing its various options and configurations, you can customize the CSV writing process to suit your specific needs. Whether you’re writing simple files or dealing with complex data structures, the csv module provides the tools you need to get the job done.